Java runtime errors occur during the execution of a Java program after the code has been compiled. These errors can be challenging to track down and may cause the program to crash.
These errors, often challenging to detect, can lead to application crashes. The post explores common causes of such errors and presents seven practical solutions to address them, empowering developers to enhance the stability and performance of their Java applications.
What are Java Runtime Errors?
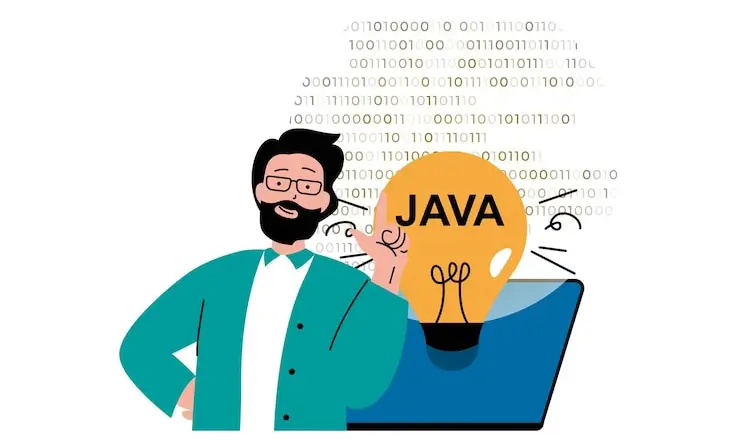
Java runtime errors are unexpected issues that arise during the execution of a Java program. These errors occur when the program violates the language’s rules and constraints, leading to abnormal conditions that the Java Virtual Machine (JVM) cannot handle.
How Java Runtime Errors Occurs?
Java runtime errors occur when a program is being executed and encounters unexpected conditions that violate the language’s rules and constraints.
Some common causes of runtime errors in Java include:
- Dividing a number by zero
- Accessing an element in an array that is out of range
- Attempting to store an incompatible type value in a collection
- Passing an invalid argument to a method
- Attempting to convert an invalid string to a number
- Insufficient space in memory for thread data
Understanding Compile-Time Errors in Java
Compile-time errors in Java are those detected by the compiler during the process of translating source code into bytecode.
Unlike runtime errors, these issues must be resolved before the program is executed.
Syntax errors, such as missing semicolons or unmatched parentheses, violate the language’s grammatical rules.
Semantic errors, involving incorrect usage of language constructs, are also flagged during compilation.
Developers depend on compiler feedback to identify and rectify these errors early in the development cycle, ensuring the generation of a valid executable and a smoother runtime experience for Java programs.
Types of Runtime Errors in Java
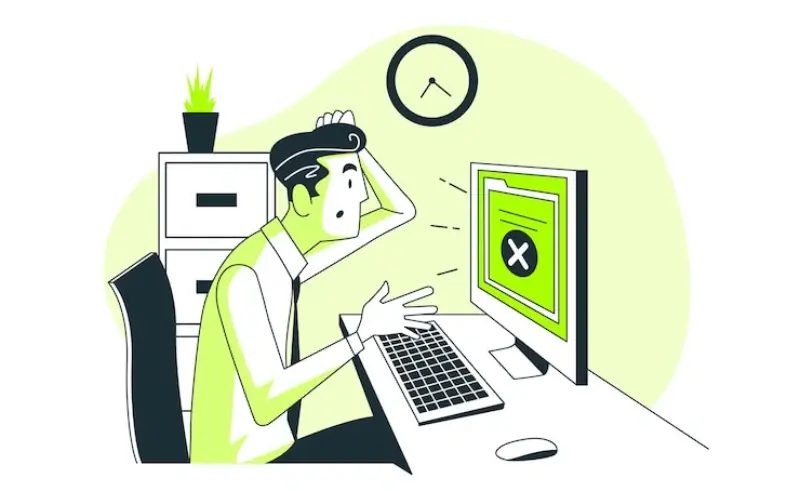
Some common types of runtime errors in Java include:
- Null Pointer Exception (NPE): This happens when a program tries to do something with an object that doesn’t exist (is null).
- ArrayIndexOutOfBoundsException: Arises when trying to access an array element using an index that is outside the bounds of the array.
- ArithmeticException: Triggered by arithmetic operations, such as division by zero, which is undefined in mathematics.
- ClassCastException: Occurs when attempting to cast an object to a class of which it is not an instance.
- ConcurrentModificationException: Arises in situations involving concurrent modification of collections, typically during iteration.
Understanding and handling these runtime errors is crucial for writing robust Java programs, and developers often use exception-handling mechanisms to manage and recover from such unexpected situations gracefully.
Common Causes of Runtime Errors in Java
Runtime errors in Java can result from a variety of causes, often stemming from unexpected or improper program behavior during execution.
Common causes include:
Null References:
Attempting to access or invoke methods on a null object reference can lead to a Null Pointer Exception.
Array Access Issues:
Accessing array elements with an index outside the valid range can trigger an ArrayIndexOutOfBoundsException.
Read more about: What is a slicer in Google Sheets
Divide by Zero:
Performing arithmetic operations, especially division, where the divisor is zero leads to an ArithmeticException.
Type Casting Problems:
Incorrectly casting objects to incompatible types can result in a ClassCastException during runtime.
Concurrent Modification:
Concurrently modifying collections, such as lists or maps, while iterating over them can cause a ConcurrentModificationException.
Resource Management:
Failing to manage resources properly, like not closing file streams or database connections, can lead to runtime errors.
Infinite Recursion:
Endless recursive calls without a proper base case can result in a StackOverflowError due to the exhaustion of the call stack.
Solution Of Java Runtime Errors
Improper validation or handling of user inputs can lead to unexpected runtime issues, such as unexpected data types or values.
- Null Check Before Access:
To address Null Pointer Exceptions, ensure that object references are properly initialized before accessing them. Implement null checks to verify that an object is not null before invoking its methods or accessing its properties.
- Array Bounds Checking:
To prevent ArrayIndexOutOfBoundsException, validate array indices before using them to access elements. Ensure that the index is within the valid range of the array length to avoid runtime errors.
- Arithmetic Operations Validation:
Guard against ArithmeticException by checking for potential division by zero scenarios before performing arithmetic operations. Utilize conditional statements to handle or prevent such situations.
- Type-Safe Casting:
To avoid ClassCastException, use the instance of the operator to check the compatibility of object types before performing casting. This helps ensure that the casting operation will not lead to runtime errors.
- Synchronized Access to Collections:
When dealing with collections in a multi-threaded environment, use proper synchronization mechanisms to prevent ConcurrentModificationException. Consider employing concurrent collections or synchronization blocks to manage concurrent access.
- Resource Cleanup:
Properly manage resources such as file streams or database connections to prevent runtime errors related to resource exhaustion. Utilize try-with-resources or ensure that resources are closed in a final block to guarantee proper cleanup.
- Input Validation and Sanitization:
To handle unexpected input issues, implement thorough validation and sanitization checks for user inputs. Verify that inputs conform to expected data types and ranges to prevent runtime errors caused by incorrect input handling.
Read more about MySQL Error 1045
Conclusion
Navigating Java Runtime Errors requires a deep understanding of their causes and effective solutions. This exploration has shed light on the diverse factors that can trigger these errors, ranging from coding issues to environmental dependencies. The seven solutions presented offer a comprehensive toolkit for developers, encompassing best practices, debugging techniques, and optimization strategies.
By embracing these solutions, developers can not only troubleshoot specific runtime errors but also cultivate a proactive mindset towards Java application development.
Still you having any errors you can get help from developers on the Java Community Tab.